1
Jul
Verify PDF signature with iText
Author: rberthou
Description
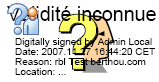
After the article “How to sign a PDF in Java” I had various demands concerning the verification of this signature.
You will find in this article an exemple of code which permit to check this.
Tools used
Exemple (source code)
As you can see the java code to do this is very simple :
package com.berthou.test.pdf ;
import java.io.*;
import java.security.*;
import java.security.cert.Certificate;
import java.util.ArrayList;
import java.util.Calendar;
import com.lowagie.text.*;
import com.lowagie.text.pdf.*;
public class test_pdf {
/**
* Name of the PDF document
*/
static String dirname = "D:\\" ;// "D:\\"
static String fname = "HelloWorld_sign" ;
public static void main(String[] args) {
try {
test_pdf.verifyPdf() ;
}
catch(Exception e) {
System.err.println(e.getMessage());
}
}
public static final boolean verifyPdf()
throws IOException, DocumentException, Exception
{
KeyStore kall = PdfPKCS7.loadCacertsKeyStore();
PdfReader reader = new PdfReader(dirname + fname +".pdf");
AcroFields af = reader.getAcroFields();
// Search of the whole signature
ArrayList names = af.getSignatureNames();
// For every signature :
for (int k = 0; k < names.size(); ++k) {
String name = (String)names.get(k);
// Affichage du nom
System.out.println("Signature name: " + name);
System.out.println("Signature covers whole document: "
+ af.signatureCoversWholeDocument(name));
// Affichage sur les revision - version
System.out.println("Document revision: " + af.getRevision(name) + " of "
+ af.getTotalRevisions());
// Debut de l'extraction de la "revision"
FileOutputStream out = new FileOutputStream("d:\\revision_"
+ af.getRevision(name) + ".pdf");
byte bb[] = new byte[8192];
InputStream ip = af.extractRevision(name);
int n = 0;
while ((n = ip.read(bb)) > 0) out.write(bb, 0, n);
out.close();
ip.close();
// Fin extraction revision
PdfPKCS7 pk = af.verifySignature(name);
Calendar cal = pk.getSignDate();
Certificate pkc[] = pk.getCertificates();
// Information about the certificat, le signataire
System.out.println("Subject: "
+ PdfPKCS7.getSubjectFields(pk.getSigningCertificate()));
// Le document à t'il ete modifié ?
System.out.println("Document modified: " + !pk.verify());
// Is the certificate avaible ? Be carefull we search the chain of certificat
Object fails[] = PdfPKCS7.verifyCertificates(pkc, kall, null, cal);
if (fails == null)
System.out.println("Certificates verified against the KeyStore");
else
System.out.println("Certificate failed: " + fails[1]);
}
return true ;
}
} |
Sample PDF generate
HelloWorld_sign.pdf
One Response pour"Verify PDF signature with iText"
Hi, Berthou, I am now supposed to implement verification of embedded PDF signatures and this article is very helpful indeed.
Well done and thanks!
Cheers
Jety
Ajouter une réponse